Properties · Methods · Examples
Declaration
TIEMask = class;
Description
Contains a selection, which is a map of selected and unselected pixels.
A selection map can have a
depth of 1 bit or 8 bit.
For a map of 1 bit, 0 is a non-selected pixel, while 1 is selected.
For a map of 8 bit, 0 is non-selected pixel, 1-254 is a "semi" selected pixel, and 255 is fully selected pixel.
TImageEnView component uses this class to store current selection in
SelectionMask property.
// Select points 50,50 and 55,55
ImageEnView1.SelectionMask.SetPixel(50, 50, 1);
ImageEnView1.SelectionMask.SetPixel(55, 55, 1);
ImageEnView1.SelectCustom();
// Exclude pixel 100,100 from selection
ImageEnView1.SelectionMask.SetPixel(100, 100, 0);
ImageEnView.SelectCustom();
// Create a new selection using only SelectionMask (this selects points 50,50 and 55,55)
ImageEnView1.SelectionMask.SetPixel(50, 50, 1);
ImageEnView1.SelectionMask.SetPixel(55, 55, 1);
ImageEnView1.SelectCustom();
// Make a gradient selection (increasing from unselected to fully selected along height of image)
// Then convert selection to gray scale
ImageEnView1.Deselect();
ImageEnView1.SelectionMaskDepth := 8;
for Y := 0 to ImageEnView1.IEBitmap.Height - 1 do
for X := 0 to ImageEnView1.IEBitmap.Width - 1 do
begin
selIntensity := Round( Y / ImageEnView1.IEBitmap.Height * 255 );
ImageEnView1.SelectionMask.SetPixel( X, Y, selIntensity );
end;
ImageEnView1.SelectCustom;
ImageEnView1.Proc.ConvertToGray();
ImageEnView1.Deselect();
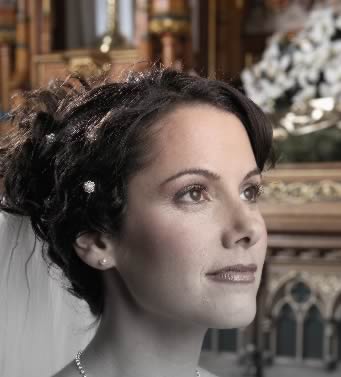
// Turn image into chessboard pattern
ImageEnView1.Deselect();
ImageEnView1.SelectionMaskDepth := 1;
for Y := 0 to ImageEnView1.IEBitmap.Height - 1 do
for X := 0 to ImageEnView1.IEBitmap.Width - 1 do
begin
if x div SQUARE_SIZE mod 2 = 0 then
sel := True
else
sel := False;
if y div SQUARE_SIZE mod 2 = 0 then
sel := not sel;
if sel then
ImageEnView1.SelectionMask.SetPixel( X, Y, 1 )
else
ImageEnView1.SelectionMask.SetPixel( X, Y, 0 );
end;
ImageEnView1.SelectCustom();
ImageEnView1.Proc.ConvertToGray();
ImageEnView1.Deselect();
// Select all pixels that are blue or green (SelectionMaskDepth=1)
for y := 0 to ImageEnView1.IEBitmap.Height - 1 do
for x := 0 to ImageEnView1.IEBitmap.Width - 1 do
begin
color := ImageEnView1.IEBitmap.Pixels[x, y];
if ( color = clBlue ) or ( color = clGreen ) then
begin
ImageEnView.SelectionMask.SetPixel(X, Y, 1);
ImageEnView.SelectCustom();
end;
end;
Properties
Methods