ImageEn, unit imageenproc |
|
TImageEnProc.ConvertTo
Declaration
procedure ConvertTo(NumColors: Integer; DitherMethod: TIEDitherMethod = ieOrdered);
function ConvertTo(PixelFormat: TIEPixelFormat; PaletteType: TIEPaletteType = ieptMedianCut; DitherType: TIEDitherType = iedtSolid; CheckParametersOnly: boolean = false): boolean;
function ConvertTo(PixelFormat: TIEPixelFormat; Palette: array of TRGB; DitherType: TIEDitherType = iedtSolid; CheckParametersOnly: boolean = false): boolean; overload;
Description
First overload reduces the number of colors in the image.
Parameter | Description |
NumColors | Number of colors of the resulting image |
DitherMethod | Color conversion algorithm to use |
Palette | Specify a custom palette (up to 256 color entries) |
When ieDithering is used and the required number of colors is <= 256 the resulting pixel format will be ie8p (unless
LegacyBitmap is true or
Location is ieTBitmap).
Second overload changes image pixel format using a combination of palette type and dithering algorithm when necessary.
This method may disable
LegacyBitmap property if necessary.
Not all combinations of parameters are possible, check the return value. Returns True on success.
Third overload forces use of a specified palette.
Parameter | Description |
PixelFormat | Required pixel format. Can be: ie1g, ie8p, ie8g, ie16g, ie24RGB, ieCMYK, ie48RGB |
PaletteType | Palette type to use when reducing colors (Do not use ieptFixedBW if you are using an ordered dithering, such as iedtOrdered8x8) |
DitherType | Dither type to use when reducing colors (If NumColors is <= 2 then only ieOrdered and ieThreshold are supported. If NumColors is > 2 then only ieDithering and ieOrdered are supported, If NumColors is > 256, then only ieOrdered is supported) |
CheckParametersOnly | If True, does not actually convert the image, just checks parameters validity |
Note:
◼If a palette is required, use
ConvertToPalette
◼Any selection is ignored. The whole image is converted to the specified palette
◼A quantizer is used to reduce the number of colors using the method specified by
ColorReductionAlgorithm
| Demos\ImageEditing\Dithering\Dithering.dpr |
| Demos\InputOutput\BatchConvert\BatchConvert.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
Method Testing
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
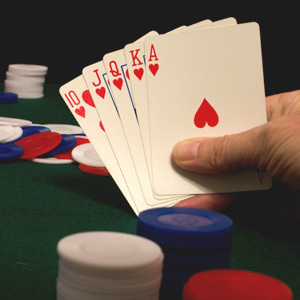
// Convert to a 4-color ordered palette
ImageEnView1.Proc.ConvertTo( 4, ieOrdered );
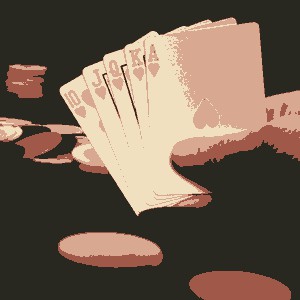
// Convert to a 4-color dithered palette
ImageEnView1.Proc.ConvertTo( 4, ieDithering );
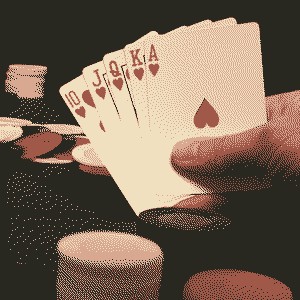
// Convert to a 16-color ordered palette
ImageEnView1.Proc.ConvertTo( 16, ieOrdered );
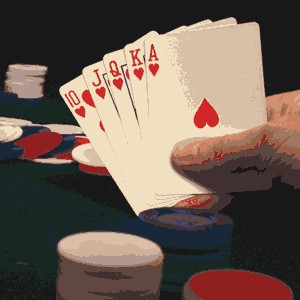
// Convert to a 16-color dithered palette
ImageEnView1.Proc.ConvertTo( 16, ieDithering );
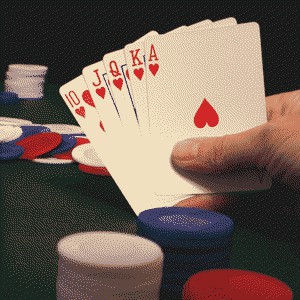
// Convert to a 64-color ordered palette
ImageEnView1.Proc.ConvertTo( 64, ieOrdered );
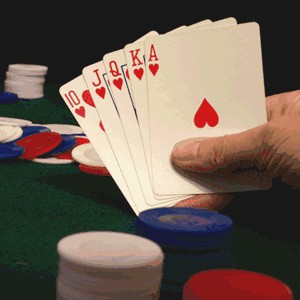
// Convert to a 64-color dithered palette
ImageEnView1.Proc.ConvertTo( 64, ieDithering );
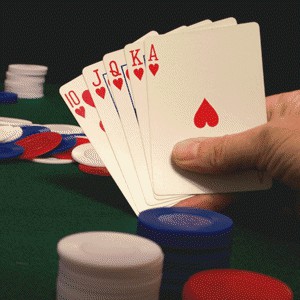
// Convert current image to 256 colors
ImageEnView1.Proc.ConvertTo(256);
// Convert current image to 461 (!) colors
ImageEnView1.Proc.ConvertTo(461);
// Convert current image to 16 colors, using Floyd-Steinberg dithering
ImageEnView1.Proc.ConvertTo(16, ieDithering);
// Convert pixel format to 256 colors with palette, using halftone palette and 8x8 dithering
ImageEnView1.Proc.ConvertTo(ie8p, ieptFixedHalftone256, iedtOrdered8x8);
// Convert pixel format to monochrome using halftone palette and 8x8 dithering
imageenview1.Proc.ConvertTo(ie1g, ieptFixedHalftone256, iedtOrdered8x8);
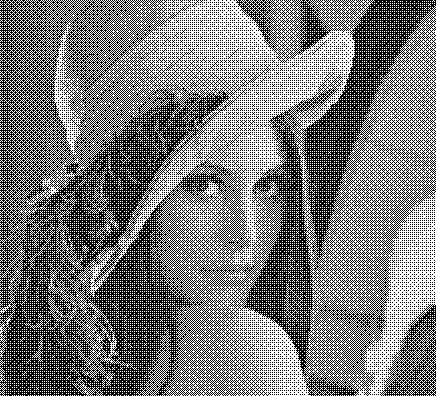
// Convert pixel format to monochrome with solid dithering
ImageEnView1.proc.ConvertTo(ie1g, ieptFixedBW, iedtSolid);
// Load a full-color image and save it as 256 color one
ImageEnView1.IO.LoadFromFile('D:\Im.jpg');
ImageEnView1.Proc.ConvertTo(ie8p, ieptFixedHalftone256, iedtOrdered8x8);
ImageEnView1.IO.Params.BitsPerSample := 8;
ImageEnView1.IO.Params.SamplesPerPixel := 1;
ImageEnView1.IO.SaveToFile('D:\Image256.bmp');
// Convert to 8 bits per pixel using the specified palette
const MYPALETTE: array [0..7] of TRGB = ((B: 0; G: 0; R:0), (B: 51; G: 0; R:0), (B: 102; G: 0; R:0), (B: 153; G: 0; R:0), (B: 204; G: 0; R:0), (B: 255; G: 0; R:0), (B: 0; G: 51; R:0), (B: 51; G: 51; R:0));
ImageEnView1.Proc.ConvertTo(ie8p, MYPALETTE, iedtErrorDiffusion);
ImageEnView1.IO.Params.BitsPerSample := 8;
ImageEnView1.IO.Params.SamplesPerPixel := 1;
ImageEnView1.IO.SaveToFile('output.png');
// Reduce image to colors within 216 color web palette (6 level RGB)
const
Color_Steps: array[0..5] of byte = (0,51,102,153,204,255);
var
Palette: array[0..215] OF TRGB;
I: Cardinal;
R,G,B: BYTE;
begin
I := LOW(Palette);
For R := 0 to 5 do
For G := 0 to 5 do
For B := 0 to 5 do
begin
Palette[ i ] := CreateRGB( Color_Steps[R], Color_Steps[G], Color_Steps[B]);
Inc(I)
end;
ImageEnView1.Proc.ConvertTo(ie8p, Palette, iedtErrorDiffusion);
end;
See Also
◼ConvertToPalette
◼BitsPerSample
◼SamplesPerPixel