ImageEn, unit imageenproc |
|
TImageEnProc.PrepareTransitionBitmaps
TImageEnProc.PrepareTransitionBitmaps
Declaration
// Standard transition overload
procedure PrepareTransitionBitmaps(StartBitmap, EndBitmap: TIEBitmap; Effect: TIETransitionType;
iWidth: Integer = -1; iHeight: Integer = -1; BackgroundColor: TColor = -1;
ResamplingFilter: TResampleFilter; Params: TIETransitionParams = nil); overload;
// Pan-Zoom overloads
procedure PrepareTransitionBitmaps(StartBitmap, EndBitmap: TIEBitmap; Effect: TIETransitionType;
StartRect, EndRect: TRect; RectMaintainAspectRatio: Boolean = True;
iWidth: Integer = -1; iHeight: Integer = -1; bStretchSmall: Boolean = False;
BackgroundColor: TColor = -1; ResamplingFilter: TResampleFilter = rfFastLinear;
Smoothing: Integer = 255; Timing: TIETransitionTiming = iettLinear); overload;
procedure PrepareTransitionBitmaps(StartBitmap, EndBitmap: TIEBitmap; Effect: TIETransitionType;
StartRect, EndRect: TRect; RectMaintainAspectRatio: Boolean;
iWidth, iHeight: Integer; bStretchSmall: Boolean;
BackgroundColor: TColor; ResamplingFilter: TResampleFilter;
Params: TIETransitionParams); overload;
Description
Use with
CreateTransitionBitmap to create a series of frames that transition from
StartBitmap to
EndBitmap.
The second overload is primarily used when creating a series of frames that show a Pan-Zoom from
StartRect to
EndRect for image
StartBitmap.
Standard Transition Overload:
Parameter | Description |
StartBitmap | The image that we transition from |
EndBitmap | The image that we transition to |
Effect | The desired transition effect |
iWidth, iHeight | The size to create the transition bitmaps. If either of these are -1 then the size will be the larger of the two images in each dimension. Aspect Ratios will be maintained and any non-image area will be filled with BackgroundColor |
BackgroundColor | The color that will be used for blank frames or non-image area (if -1 then Background is used) |
ResamplingFilter | The algorithm that is used to improve quality when resizing images |
Params | Extra transition properties |
Pan-Zoom Overloads:
Parameter | Description |
StartBitmap | The image that we transition from |
EndBitmap | The image that we transition to (NIL for a iettPanZoom transition) |
Effect | The desired transition effect |
StartRect | When using an iettPanZoom effect this is the portion of the image that is shown at the start |
EndRect | When using an iettPanZoom effect this is the portion of the image that is shown at the end |
RectMaintainAspectRatio | ImageEn will ensure that the starting and ending rects are automatically adjusted to ensure the resultant image has the correct aspect ratio (iettPanZoom only) |
iWidth, iHeight | The size to create the transition bitmaps. If either of these are -1 then the size will be the larger of the two images in each dimension. Aspect Ratios will be maintained and any non-image area will be filled with BackgroundColor |
bStretchSmall | If the images are smaller than the transition bitmap size (iWidth x iHeight) should they be stretched to fit (which can lead to distortion) |
BackgroundColor | The color that will be used for blank frames or non-image area (if -1 then Background is used) |
ResamplingFilter | The algorithm that is used to improve quality when resizing images |
Smoothing | In order to reduce the "jumpiness" of pan-zoom effects, transition frames can be alpha blended. A low value will improve smoothness, but increase blurriness. A high value will improve clarity, but increase jumpiness. Typical range is 64 - 196. 255 means no alpha blending (which is best when outputting to a bitmap) |
Timing | The rate at which the transition progresses |
Params | Extra transition properties |
To create Pan-Zoom transitions for an image call it as follows:
PrepareTransitionBitmaps(MyBitmap, nil, iettPanZoom, StartingRect, EndingRect);
CreateTransitionBitmap(TransitionLevel, MyPanZoomBitmap);
Note:
◼This is only for creating transition bitmaps for external purposes. To show an image with a transition effect in ImageEn, use
RunTransition
◼You will need to add iexTransitions to your uses clause to access the transition types
| Demos\Multi\CreateTransitionFrames\CreateTransitionFrames.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
procedure TransitionFrameCreationExample();
var
OldBitmap, NewBitmap, TransBitmap: TBitmap;
i: Integer;
TransLevel: Single;
begin
OldBitmap := TBitmap.Create;
NewBitmap := TBitmap.Create;
TransBitmap := TBitmap.Create;
try
OldBitmap.LoadFromFile('C:\OldImage.bmp');
NewBitmap.LoadFromFile('C:\NewImage.bmp');
// Call PrepareTransitionBitmaps once
ImageEnProc.PrepareTransitionBitmaps(OldBitmap, NewBitmap, iettCrossDissolve);
for i := 1 to 9 do
begin
// Transition levels from 10% to 90%
TransLevel := i * 10;
// Call CreateTransitionBitmap for each required frame
ImageEnProc.CreateTransitionBitmap(TransLevel, TransBitmap);
TransBitmap.SaveToFile('C:\TransImage' + IntToStr(I) + '.bmp');
end;
finally
OldBitmap.Free;
NewBitmap.Free;
TransBitmap.Free;
end;
end;
procedure PanZoomFrameCreationExample(StartingRect, EndingRect : TRect);
var
MyBitmap, PanZoomBitmap : TBitmap;
I : Integer;
TransLevel : Single;
begin
MyBitmap := TBitmap.Create;
PanZoomBitmap := TBitmap.Create;
try
MyBitmap.LoadFromFile('C:\MyImage.bmp');
// Call PrepareTransitionBitmaps once
ImageEnProc.PrepareTransitionBitmaps(MyBitmap, MyBitmap, iettPanZoom, StartingRect, EndingRect);
for i := 0 to 10 do
begin
// Pan-Zoom Transitions from StartingRect (0%) to EndingRect (100%)
TransLevel := i * 10;
// Call CreateTransitionBitmap for each required frame
ImageEnProc.CreateTransitionBitmap(TransLevel, PanZoomBitmap);
PanZoomBitmap.SaveToFile('C:\PanZoomImage' + IntToStr(I) + '.bmp');
end;
finally
MyBitmap.Free;
PanZoomBitmap.Free;
end;
end;
// Random transition from fully black to an image
bmpOut := TIEBitmap.Create( 'D:\TestImage.jpg' );
bmpIn := TIEBitmap.Create( bmpOut.Width, bmpOut.Height, clBlack );
// Prepare transition
trans := TIETransitionType( 1 + Random( ord( High( TIETransitionType )) - 2 )); // Random transition
ImageEnView1.Proc.PrepareTransitionBitmaps( bmpIn, bmpOut, trans );
// Get transition image
ImageEnView1.Proc.CreateTransitionBitmap( percentage, ImageEnView1.IEBitmap );
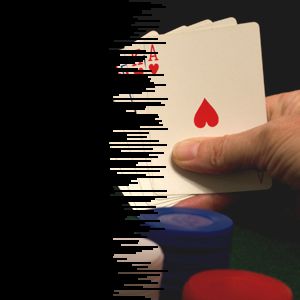
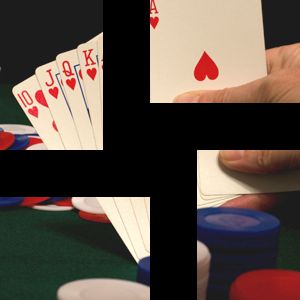
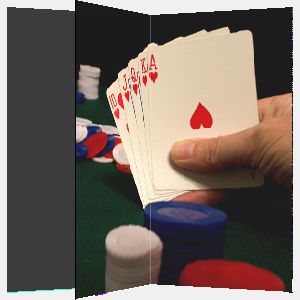
// Create an AVI transitioning one image to another
const
Frames_Per_Second = 20;
Display_Seconds = 5;
var
proc: TImageEnProc;
io: TImageEnIO;
startBitmap, endBitmap : TIEBitmap;
i, frameCount: Integer;
transLevel: Single;
begin
startBitmap := TIEBitmap.Create();
endBitmap := TIEBitmap.Create();
proc := TImageEnProc.Create( nil );
io := TImageEnIO.Create( nil );
try
if io.CreateAVIFile('D:\Transition.avi', Frames_Per_Second, 'cvid' ) = ieaviNOCOMPRESSOR then
raise Exception.create( 'This compressor is unavailable!' );
startBitmap.LoadFromFile( 'D:\image1.jpg' );
endBitmap.LoadFromFile( 'D:\image2.jpg' );
// Call PrepareTransitionBitmaps once
proc.PrepareTransitionBitmaps(startBitmap, endBitmap, SelectedTransitionEffect);
frameCount := Display_Seconds * Frames_Per_Second;
for i := 0 to frameCount - 1 do
begin
// We want levels from 0% to 100% (show start and end)
transLevel := 100 / ( frameCount - 1 ) * i;
// Call CreateTransitionBitmap for each required frame
proc.CreateTransitionBitmap( transLevel, io.IEBitmap );
io.SaveToAVI();
Caption := IntToStr( Round( i / frameCount * 100 )) + '%';
end;
io.CloseAVIFile();
Caption := 'Done!';
finally
startBitmap.Free();
endBitmap.Free();
proc.Free();
io.Free();
end;
end;
See Also
◼CreateTransitionBitmap