Properties · Methods · Demos · Examples
Declaration
TIEPolylineLayer = class(TIELayer);
Description
TImageEnView supports multiple layers, allowing the creation of a single image from multiple source images (which can be resized, rotated, moved, etc).
TIEPolylineLayer is a descendent of
TIELayer that displays a line formed from multiple points. It can also be closed to display a polygon.
You can easily add curved lines to polyline layers:
You can create polyline layers with code using
LayersAdd or by user action by setting
MouseInteractLayers:
Item | Description |
mlClickCreatePolylineLayers | Click multiple points to create a polyline layer * |
mlDrawCreatePolylineLayers | Click and drag to freehand draw a polyline layer * |
mlEditLayerPoints | Click and drag points to move them. Click on the polyline to add a point, Alt key to convert a point to a curve, Ctrl+click or delete key to remove a point |
mlCreatePolylineLayers | Drag the area of a polyline layer (you must then use code to add points) |
* Auto-closing of the polyline layer is controlled by LayersAutoClosePolylines
Review the
Layer Documentation for a full description of layer support.
Demo | Description | Demo Project Folder | Compiled Demo |
Line Layer Editing | Creating and point editing line, poly-line and angle layers | LayerEditing\Layers_Lines\Layers.dpr | |
All Layer Editing | Usage of image, shape, text, polygon and line layers | LayerEditing\Layers_AllTypes\Layers.dpr | |
Magic Fill to Polygon | Creates a polygon (closed TIEPolylineLayer) by performing a magic selection on an image (matching color range) | LayerEditing\MagicFillToPolygon\Magic2Polygon.dpr | |
// Draw a triangle polygon
With TIEPolylineLayer( ImageEnView1.CurrentLayer ) do
begin
ClearAllPoints();
AddPoint( 500, 0 );
AddPoint( 1000, 1000 );
AddPoint( 0, 1000 );
PolylineClosed := True;
end;
ImageEnView1.Update();
// Which is the same as..
var
shapePoints: array of TPoint;
begin
SetLength( shapePoints, 3 );
shapePoints[0] := Point( 500, 0 );
shapePoints[1] := Point( 1000, 1000 );
shapePoints[2] := Point( 0, 1000 );
TIEPolylineLayer( ImageEnView1.CurrentLayer ).SetPoints( shapePoints, True );
ImageEnView1.Update();
end;
// Which is the same as...
TIEPolylineLayer( ImageEnView1.CurrentLayer ).SetPoints( [ Point(500, 0), Point(1000, 1000), Point(0, 1000) ], True );
ImageEnView1.Update();
// Which is the same as...
TIEPolylineLayer( ImageEnView1.CurrentLayer ).SetPoints( iesTriangle, True );
ImageEnView1.Update();
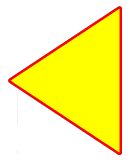
// If PolylineClosed is not enabled, we get a polyline, instead of a polygon
TIEPolylineLayer( ImageEnView1.CurrentLayer ).PolylineClosed := False;
ImageEnView1.Update();
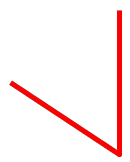
// Allow users to create and edit a polygon
ImageEnView1.LayersAutoClosePolylines := iecmAlways;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers, mlEditLayerPoints ];
// Allow users to freehand draw a polyline
ImageEnView1.MouseInteractLayers := [ mlDrawCreatePolylineLayers, mlEditLayerPoints ];
// Allow users to create and edit a polyline
ImageEnView1.LayersAutoClosePolylines := iecmManual;
ImageEnView1.MouseInteractLayers := [ mlClickCreatePolylineLayers, mlEditLayerPoints ];
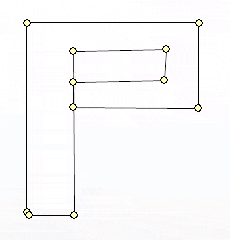
Hold Alt to convert a line to a curve...
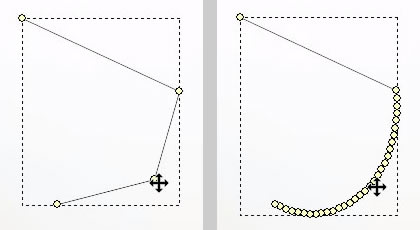
// Add a 5-pointed star polyline layer
var
pp: array[0..9] of TPoint;
polyLayer: TIEPolylineLayer;
begin
pp[0] := Point(175, 50);
pp[1] := Point(205, 145);
pp[2] := Point(300, 145);
pp[3] := Point(225, 205);
pp[4] := Point(253, 300);
pp[5] := Point(175, 243);
pp[6] := Point(98 , 300);
pp[7] := Point(128, 205);
pp[8] := Point(50 , 145);
pp[9] := Point(148, 145);
ImageEnView1.LayersAdd( ielkPolyline );
polyLayer := TIEPolylineLayer( ImageEnView1.CurrentLayer );
polyLayer.LineColor := clOrangeRed;
polyLayer.LineWidth := 3;
polyLayer.FillColor := clYellow;
polyLayer.SetPoints( pp, True, iepbBitmap );
ImageEnView1.Update();
end;
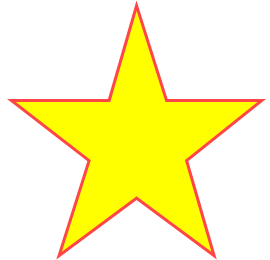
// Draw a pink balloon
ImageEnView1.LayersAdd( ielkPolyline, 100, 100, 600, 800 );
With TIEPolylineLayer( ImageEnView1.CurrentLayer ) do
begin
AddPoint( 500, 500 );
AddCurvePoints( 0.95, Point( 500, 0 ));
AddCurvePoints( 0.95, Point( 500, 500 ));
AddPoint($FFFEE, $FFFEE); // End shape and close polyline
AddPoint( 500, 500 );
AddPoint( 520, 517 );
AddPoint( 480, 517 );
AddPoint($FFFEE, $FFFEE); // End shape and close polyline
AddPoint( 500, 517 );
AddCurvePoints( 3, Point( 500, 690 ));
AddCurvePoints( -3, Point( 510, 850 ));
AddCurvePoints( 3, Point( 500, 1000 ));
AddPoint($FFFFF, $FFFFF); // End polyline (optional at end)
LineWidth := 2;
FillColor := $00921CF0;
PolylineClosed := False;
end;
ImageEnView1.Update();
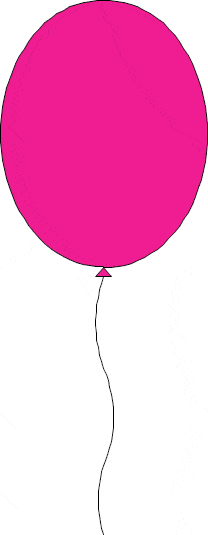
General
Polygon Points
Style
Size and Position
Unique to TIEPolylineLayer
See Also
◼Layer Editing Overview
◼LayersAdd
◼LayersInsert
◼MouseInteractLayers
◼AdvancedDrawPolyline